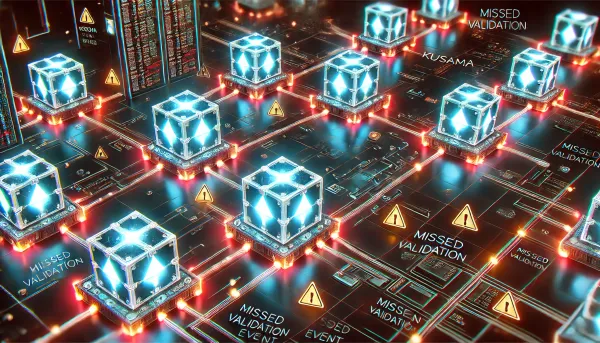
Kusama: missed Paravalidation vote investigation
It finally happened. My first ever "incident" during Kusama para-validation (missed vote). Let me explain what happened, how I noticed it and what investigations I conducted to understand the reason behind it. Spoiler: the impact of this is negligible either on the network or the staking rewards. Turns